W tym poście chciałbym przedstawić sposób wykonania aplikacji odczytującej pogode z serwisu openWeatherApp za pomocą JavaScrip oraz jQuerry.
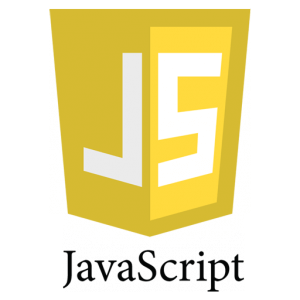
[Źródło: www.javatpoint.com]
Strona HTML:
Kod HTML:
- <!Doctype html>
- <html>
- <head>
- <title>Get weather from openWeatherApp</title>
- <link rel="stylesheet" type="text/css" href="css/style.css">
- <script src="http://code.jquery.com/jquery-2.1.4.min.js" ></script>
- <script src="js/script.js" ></script>
- </head>
- <body>
- <div>
- <h1>Get weather from openWeatherApp</h1>
- </div>
- <div class="header">
- <a class="button" href="index.html">Main page</a>
- <select id = "select">
- <option class=".dropBoxStyle" value="all">Get all data</option>
- <option class=".dropBoxStyle" value="tempPress">Temperature and pressure</option>
- <option class=".dropBoxStyle" value="cloudsWind">Clouds and wind</option>
- <option class=".dropBoxStyle" value="sunSetRise">Sunset and sunrise</option>
- <option class=".dropBoxStyle" value="coords">Coordinates</option>
- </select>
- <input type="text" id="input">
- <button type="button" id="submit">Get Data</button>
- <script src="js/btnClick.js" ></script>
- </div>
- <div id="content" class="weatherInfo-list">
- </div>
- </body>
- </html>
Przygotowana strona jest dosyć prosta. Znajduje się tam nagłówek, przycisk do przechodzenia na główną stronę, rozwijalny wybór odczytywanych informacji oraz przycisk wyszukiwania.
Wygląd strony jest następujący:
Strona z załadowanymi informacjami o pogodzie:
Kolejne miasta ładowane są na pierwszej pozycji (tzn. od lewej). Wcześniejsze odczytane pozycje są przesuwane.
Wszystkie style zostały zdefiniowane w pliku CSS, który można pobrać z dysku google. Link na dole strony.
JavaScript:
Kod JavaScript został rozłożony w dwóch plikach. Jeden odpowiada za załadowanie aplikacji pogodowej. Drugi natomiast obsługuje wciśnięcie klawisza Enter w celu załadowania danych.
Odczyt danych z serwisu pogodowego:
- $(document).ready(function(){
- $('#submit').click(function(){
- var cityName=$('#input').val();
- var weatherURL="http://api.openweathermap.org/data/2.5/weather?q="+cityName+"&units=metric&appid=<<APPID_KEY>>";
- $.ajax({
- url:weatherURL,
- success:function(result){
- var html;
- var type = $('#select').val();
- var label = $('#select option:selected').text();
- switch(type)
- {
- /* -------------------------------------------------------------------------------- */
- case 'all':
- html =
- openUnorderedList() +
- prepareWeatherIconAndDescription(result.weather) +
- prepareStringWithCountry(result.sys.country) +
- prepareStringWithTemp(result.main.temp, result.main.temp_min, result.main.temp_max) +
- prepareStringWithPress(result.main.pressure, result.main.sea_level) +
- prepareStringWithWindDegre(result.wind.deg) +
- prepareStringWithWindSpeed(result.wind.speed) +
- prepareStringWithSunRiseTime(result.sys.sunrise) +
- prepareStringWithSunSetTime(result.sys.sunset) +
- prepareStringWithCoordLong(result.coord.lon) +
- prepareStringWithCoordLat(result.coord.lat) +
- closeUnorderedList();
- break;
- /* -------------------------------------------------------------------------------- */
- case 'tempPress':
- html =
- openUnorderedList() +
- prepareStringWithTemp(result.main.temp, result.main.temp_min, result.main.temp_max) +
- prepareStringWithPress(result.main.pressure, result.main.sea_level) +
- closeUnorderedList();
- break;
- /* -------------------------------------------------------------------------------- */
- case 'cloudsWind':
- html =
- openUnorderedList() +
- prepareWeatherIconAndDescription(result.weather) +
- prepareStringWithWindDegre(result.wind.deg) +
- prepareStringWithWindSpeed(result.wind.speed) +
- closeUnorderedList();
- break;
- /* -------------------------------------------------------------------------------- */
- case 'sunSetRise':
- html =
- openUnorderedList() +
- prepareStringWithSunRiseTime(result.sys.sunrise) +
- prepareStringWithSunSetTime(result.sys.sunset) +
- closeUnorderedList();
- break;
- /* -------------------------------------------------------------------------------- */
- case 'coords':
- html =
- openUnorderedList();
- prepareStringWithCoordLong(result.coord.lon) +
- prepareStringWithCoordLat(result.coord.lat) +
- closeUnorderedList();
- break;
- /* -------------------------------------------------------------------------------- */
- default:
- return;}
- $('#content').prepend(
- wrapNewDataPage(html, result.sys.country, result.name)
- );
- }
- })
- })
- });
- // ----------------------------------------------------------------------------- //
- function convertFromUnixTime(unixTime) {
- var date = new Date(unixTime * 1000);
- var hours = date.getHours();
- var minutes = "0" + date.getMinutes();
- var seconds = "0" + date.getSeconds();
- var formattedTime = hours + ':' + minutes.substr(-2) + ':' + seconds.substr(-2);
- return formattedTime;
- }
- // ----------------------------------------------------------------------------- //
- function openUnorderedList(){
- return ' <ul>';
- }
- // ----------------------------------------------------------------------------- //
- function closeUnorderedList(){
- return ' </ul>';
- }
- // ----------------------------------------------------------------------------- //
- function prepareStringWithTemp(temp, tempMin, tempMax){
- return ' <li><b>Temperature:</b> ' + temp + ' <em>(min: ' + tempMin + ', max: ' + tempMax + ')</em></li>';
- }
- // ----------------------------------------------------------------------------- //
- function prepareStringWithPress(pressure, seaLevel){
- return ' <li><b>Presure:</b> ' + pressure + ' hPa <em>(sea: ' + seaLevel+ ')</em></li>';
- }
- // ----------------------------------------------------------------------------- //
- function prepareStringWithWindDegre(windDeg){
- return ' <li><b>Wind degrees:</b> ' + windDeg + '</li>';
- }
- // ----------------------------------------------------------------------------- //
- function prepareStringWithWindSpeed(windSpeed){
- return ' <li><b>Speed:</b> ' + windSpeed + '</li>';
- }
- // ----------------------------------------------------------------------------- //
- function prepareStringWithCoordLong(coordLong){
- return ' <li><b>Longitude:</b> ' + coordLong + '</li>';
- }
- // ----------------------------------------------------------------------------- //
- function prepareStringWithCoordLat(coordLat){
- return ' <li><b>Latitude:</b> ' + coordLat + '</li>';
- }
- // ----------------------------------------------------------------------------- //
- function prepareStringWithSunRiseTime(unixTime){
- var sunRiseTime = convertFromUnixTime(unixTime);
- return ' <li><b>Sunrise:</b> ' + sunRiseTime + '</li>';
- }
- // ----------------------------------------------------------------------------- //
- function prepareStringWithSunSetTime(unixTime){
- var sunRiseTime = convertFromUnixTime(unixTime);
- return ' <li><b>Sunset:</b> ' + sunRiseTime + '</li>';
- }
- // ----------------------------------------------------------------------------- //
- function prepareWeatherIconAndDescription(weather){
- var html;
- $.each(weather, function(key, weather) {
- html =
- '<li><img class="weatherInfo-icon" src="http://openweathermap.org/img/w/' + weather.icon + '.png" /><b>' + weather.main +'</b></li>' +
- '<li><b>Description:</b> ' + weather.description + '</li>';
- });
- return html;
- }
- // ----------------------------------------------------------------------------- //
- function prepareStringWithCountry(countryId){
- switch(countryId)
- {
- case 'GB':
- return ' <li><b>Country:</b> Great Britain</li>';
- case 'PL':
- return ' <li><b>Country:</b> Poland</li>';
- case 'US':
- return ' <li><b>Country:</b> United States</li>';
- default:
- return ' <li><b>Country:</b> ' + countryId + '</li>';
- }
- }
- // ----------------------------------------------------------------------------- //
- function wrapNewDataPage(html, country, resultName){
- return '<article class="weatherInfo">' +
- ' <h2>' + country + " - " + resultName + '</h2>' +
- ' <div class="weatherInfo-main">' +
- html +
- ' </div>' +
- '</article>';
- }
Do odczytywania danych obsługuje z serwera wykorzystuje metodę ajax. Pozwala ona na odczytanie danych z serwera. Gdy ta operacja się powiedzie, funkcja przygotowuje dane do wyświetlenia bazując na informacjach przekazanych z głównej strony. Każda z informacji jest obrabiana w osobnej funkcji.
Obsługa klawisza enter:
- var input = document.getElementById("input");
- input.addEventListener("keyup", function(event)
- {
- event.preventDefault();
- if (event.keyCode === 13) {
- document.getElementById("submit").click();
- }
- });
Na samym początku pobierany jest kod wciśniętego klawisza. Następnie sprawdzam czy został wciśnięty klawisz enter. Jeśli tak to następuje wyzwolenie wciśnięcia klawisza pobrania danych.
Wszystkie pliki można pobrać z dysku Google pod tym linkiem.