W tym poście chciałbym opisać krótką bibliotekę pozwalająca na zapis bądź odczyt danych z serwisu thingspeak.
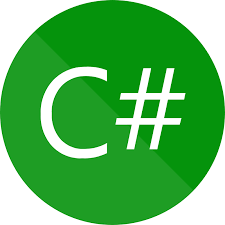
[Źródło: https://docs.microsoft.com/en-us/dotnet/]
Biblioteka:
Dane z serwisu można pobierać poprzez przesłanie odpowiedniego żądania ze strony internetowej. Odczytane dane przesłane są w formacie JSON.
W celu wprowadzenia danych należy wysłać następujące żądanie:
W celu wprowadzenia danych należy wysłać następujące żądanie:
- https://api.thingspeak.com/update?api_key=<WRITE_KEY>&field1=<VALUE_TO_WRITE>
Jako odpowiedź przesłana będzie liczba oznaczająca numer aktualizacji danych.
Gdy chcemy odczytać dane to można użyć jednej z dwóch opcji.
Odczyt zdefiniowanej liczby przesłanych danych (Read Channel Feed):
- https://api.thingspeak.com/channels/<CHANNEL_ID>/feeds.json?api_key=<READ_KEY>&results=<NUMBER_OF_DATA>
Otrzymane dane w formacie JSON wyglądają następująco:
- {
- "channel":
- {
- "id":807800,
- "name":"ESP32_DHT",
- "latitude":"0.0",
- "longitude":"0.0",
- "field1":"Temp_DHT",
- "field2":"Humi_DHT",
- "created_at":"2019-06-23T15:03:48Z",
- "updated_at":"2019-06-23T15:04:10Z",
- "last_entry_id":3484
- },
- "feeds":[
- {
- "created_at":"2020-01-31T19:49:41Z",
- "entry_id":3483,
- "field1":"0",
- "field2":null
- },
- {
- "created_at":"2020-01-31T20:04:02Z",
- "entry_id":3484,
- "field1":"0",
- "field2":null
- }
- ]
- }
Odczyt zdefiniowanej liczby danych z danego pola (Read Channel Field):
- https://api.thingspeak.com/channels/<CHANNEL_ID>/fields/<FIELD_ID>.json?api_key=<READ_KEY>&results=<NUMBER_OF_DATA_TO_READ>
Odczytane dane wyglądają następująco:
- {
- "channel:
- {
- "id":807800,
- "name":"ESP32_DHT",
- "latitude":"0.0",
- "longitude":"0.0",
- "field1":"Temp_DHT",
- "field2":"Humi_DHT",
- "created_at":"2019-06-23T15:03:48Z",
- "updated_at":"2019-06-23T15:04:10Z",
- "last_entry_id":3484
},
- "feeds":
- [
- {
- "created_at":"2020-01-31T19:49:41Z",
- "entry_id":3483,
- "field1":"0"
- },
- {
- "created_at":"2020-01-31T20:04:02Z",
- "entry_id":3484,
- "field1":"0"
- }
- ]
- }
- }
Ostatnia opcja pozwala na przeglądnięcie statusu aktualizacji danych.
- https://api.thingspeak.com/channels/<CHANNEL_ID>/status.json?api_key=<READ_KEY>
Odebrany JSON dla powyższego zapytania wygląda następująco:
- {
- "channel":
- {
- "name":"ESP32_DHT",
- "latitude":"0.0",
- "longitude":"0.0"
- },
- "feeds":
- [
- {
- "created_at":"2020-01-31T19:46:13Z",
- "entry_id":3480,"status":null
- },
- {
- "created_at":"2020-01-31T19:48:21Z",
- "entry_id":3481,"status":null
- },
- {
- "created_at":"2020-01-31T19:49:02Z",
- "entry_id":3482,
- "status":null
- },
- {
- "created_at":"2020-01-31T19:49:41Z",
- "entry_id":3483,
- "status":null
- },
- {
- "created_at":"2020-01-31T20:04:02Z",
- "entry_id":3484,
- "status":null
- }
- ]
- }
Aby wysłać i odebrać dane należy posłużyć się następującymi funkcjami:
Pierwsze cztery funkcje przygotowują string z żądaniem, który należy podać jako parametr funkcji ostatniej gdzie wysyłana jest informacja do serwera a po niej następuje odebranie danych w formacie JSON. Funkcja zwraca cały odebrany obiekt.
- public string GetUpdateDataString(byte fieldNumber, int valueToWrite)
- {
- return strUpdateBase + " ? key=" + _writeKey + "&field" + fieldNumber.ToString() + "=";
- }
- public string GetReadFeedString(byte numberOfDataToRead)
- {
- return strReadData + _channelId +
- strReadFeed_SecondPart + _readKey + "&results" + "=" + numberOfDataToRead;
- }
- public string GetReadFieldString(byte fieldNumber, byte numberOfDataToRead)
- {
- return strReadData + _channelId +
- strFields + fieldNumber.ToString() + strFields_2 + _readKey + "&results" + "=" + numberOfDataToRead;
- }
- public string GetReaddUpdateHistoryString(string channelID)
- {
- return strReadData + _channelId + "/status.json?api_key=" + _readKey;
- }
- public string SendRequest(string requestToSend)
- {
- string readedData = "";
- try
- {
- HttpWebRequest ThingsSpeakReq;
- HttpWebResponse ThingsSpeakResp;
- ThingsSpeakReq = (HttpWebRequest)WebRequest.Create(requestToSend);
- ThingsSpeakReq.Method = WebRequestMethods.Http.Get;
- ThingsSpeakReq.Accept = "application/json";
- ThingsSpeakResp = (HttpWebResponse)ThingsSpeakReq.GetResponse();
- using (Stream responseStream = ThingsSpeakResp.GetResponseStream())
- {
- StreamReader reader = new StreamReader(responseStream, Encoding.UTF8);
- readedData = (reader.ReadToEnd());
- }
- if (!(string.Equals(ThingsSpeakResp.StatusDescription, "OK")))
- {
- Exception exData = new Exception(ThingsSpeakResp.StatusDescription);
- return exData.ToString();
- }
- }
- catch (Exception ex)
- {
- return ex.ToString();
- }
- return readedData;
- }
Odebrane dane w formacie JSON można obrobić za pomocą biblioteki np. Newtonsoft.Json.
Cała klasa wygląda następująco:
- class ThingspeakLib
- {
- string _writeKey = "";
- string _readKey = "";
- string _channelId = "";
- readonly string strUpdateBase = "http://api.thingspeak.com/update";
- readonly string strReadData = "https://api.thingspeak.com/channels/";
- readonly string strReadFeed_SecondPart = @"/feeds.json?api_key=";
- readonly string strFields = @"/fields/";
- readonly string strFields_2 = ".json?api_key=";
- readonly string strStatusUpdates = @"/status.json?api_key=";
- public string WriteKey
- {
- get { return _writeKey; }
- }
- public string ReadKey
- {
- get { return _readKey; }
- }
- public string ChannelId
- {
- get { return _channelId; }
- }
- public void SetWriteKey(string keyVal)
- {
- writeKey = keyVal;
- }
- public void SetReadKey(string keyVal)
- {
- readKey = keyVal;
- }
- public void SetChannelId(string channelIdVal)
- {
- _channelId = channelIdVal;
- }
- public string GetUpdateDataString(byte fieldNumber, int valueToWrite)
- {
- return strUpdateBase + " ? key=" + _writeKey + "&field" + fieldNumber.ToString() + "=";
- }
- public string GetReadFeedString(byte numberOfDataToRead)
- {
- return strReadData + _channelId +
- strReadFeed_SecondPart + _readKey + "&results" + "=" + numberOfDataToRead;
- }
- public string GetReadFieldString(byte fieldNumber, byte numberOfDataToRead)
- {
- return strReadData + _channelId +
- strFields + fieldNumber.ToString() + strFields_2 + _readKey + "&results" + "=" + numberOfDataToRead;
- }
- public string GetReaddUpdateHistoryString(string channelID)
- {
- return strReadData + _channelId + "/status.json?api_key=" + _readKey;
- }
- public string SendRequest(string requestToSend)
- {
- string readedData = "";
- try
- {
- HttpWebRequest ThingsSpeakReq;
- HttpWebResponse ThingsSpeakResp;
- ThingsSpeakReq = (HttpWebRequest)WebRequest.Create(requestToSend);
- ThingsSpeakReq.Method = WebRequestMethods.Http.Get;
- ThingsSpeakReq.Accept = "application/json";
- ThingsSpeakResp = (HttpWebResponse)ThingsSpeakReq.GetResponse();
- using (Stream responseStream = ThingsSpeakResp.GetResponseStream())
- {
- StreamReader reader = new StreamReader(responseStream, Encoding.UTF8);
- readedData = (reader.ReadToEnd());
- }
- if (!(string.Equals(ThingsSpeakResp.StatusDescription, "OK")))
- {
- Exception exData = new Exception(ThingsSpeakResp.StatusDescription);
- return exData.ToString();
- }
- }
- catch (Exception ex)
- {
- return ex.ToString();
- }
- return readedData;
- }
- }